and share code with your internal team
Prerequisites
This is a recipe to use git and npm to distribute your code in your internal team. This will not teach you about Git or NPM.
you must know about Git, tags, npm and their configuration files.
Context
npm allows distributing and sharing code between developers, the usual way to share the code is creating a package.json and with npm publish you can easily share the code with the world, but what happens in case you only want to share the code with a small group of people? In my little experience I know 2 possibilities.
- Using scope packages or
- Using git with tags
For solution 1, you have to pay for the service and you can find documentation in https://docs.npmjs.com/creating-and-publishing-private-packages.
As an alternative you can use a git repository no matter its location (github, bitbucket, your own server, etc) and using npm you can distribute the code. In this case only people with access to the git repository can install and update the package.
The implementation we will check it’s about this second solution.
Implementation
Setup your repository
There is nothing special in this step, you only need to create a repo in the place you consider i.e github, bitbucket, etc, but the key concept here, is the security, remember if a person has access to the repo, that person will be able to install the package.
Create a package.json
It’s really easy to create a package.json, you can create the file manually with the right structure, or even easier you can execute the following command
npm init
You will be guided step by step with questions such as the name of the project, main entry point, etc.
About package versions
Once you have the package.json and you check the content of the file, you will note a particular attribute called “version”, this version must follow the semver format and this looks like 1.0.0, you can get more information in https://semver.org/
This version is really important because it is a standard and your clients (internal team using your package in other projects) can define what updates they want to include.
For example, It’s usually common for you clients to include all the changes from an specific major release, let’s imagine a client want all the changes from version 1.2.0 and future minor changes, in this particular case your client will reference your package with the following semver pattern ^1.2 and in theory if you respect the semver philosophy, your client won’t have problems executing npm update keeping backward compatibility. you can get more detail in https://docs.npmjs.com/about-semantic-versioning
Creating git tags
With git tags (or branches) you can tell to your public where the commits with specific versions are, if you say in the package.json your version is 1.0.0 , you should create a tag with this name 1.0.0 at that specific commit. you can check the documentation about tags in https://git-scm.com/book/en/v2/Git-Basics-Tagging
Test the package
For an exercise we can create a test project using the cowsay package. you usually just need to run node install cowsay, but for the exercise we will install the package from the github repository.
cowsay will print a cow with the text that you want.
Init the project
- Create a folder called test
- Run npm init and accept all default options
- npm has created a file package.json with the following content
{
"name": "test",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC"
}
Install cowsay from repository
repository of the cowsay is located in https://github.com/piuccio/cowsay, and the current tags at the moment to write this recipe are:
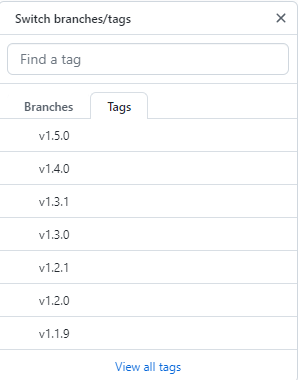
so, suppose for our project we only want to work with the version 1.2 and all the future fixes, that means our expression of semver should be 1.2.x, so npm will install v1.2.1 and in case of future fix releases and executing npm update, system will take in account those changes (as 1.2.3, 1.2.4, 1.2.5, and so on).
to achieve the previous objective we need to run the following command
npm install https://github.com/piuccio/cowsay#semver:1.2.x
now, you can check the package.json and you will find the following lines:
"dependencies": {
"cowsay": "github:piuccio/cowsay#semver:1.2.x"
}
and in case you check the package.lock.json which is the file with all the specific version installed, you will see the following lines regarding the cowsay:
"node_modules/cowsay": {
"version": "1.2.1",
"resolved": git+ssh://git@github.com/piuccio/cowsay.git#e3ecdc5e07558afc33a2f17ff8cc450fc306af4f",
"license": "MIT",
"dependencies": {
"get-stdin": "^5.0.1",
"optimist": "~0.6.1",
"string-width": "~2.1.1"
}
as you can see the latest version that applies the pattern 1.2.x is currently installed which is the version 1.2.1.
using cowsay
from a terminal in the root of your test project execute the following command
> npx cowsay hello
and you will get
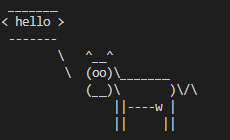
Leave a Reply